【Go】GOでPostgresqlへ接続する
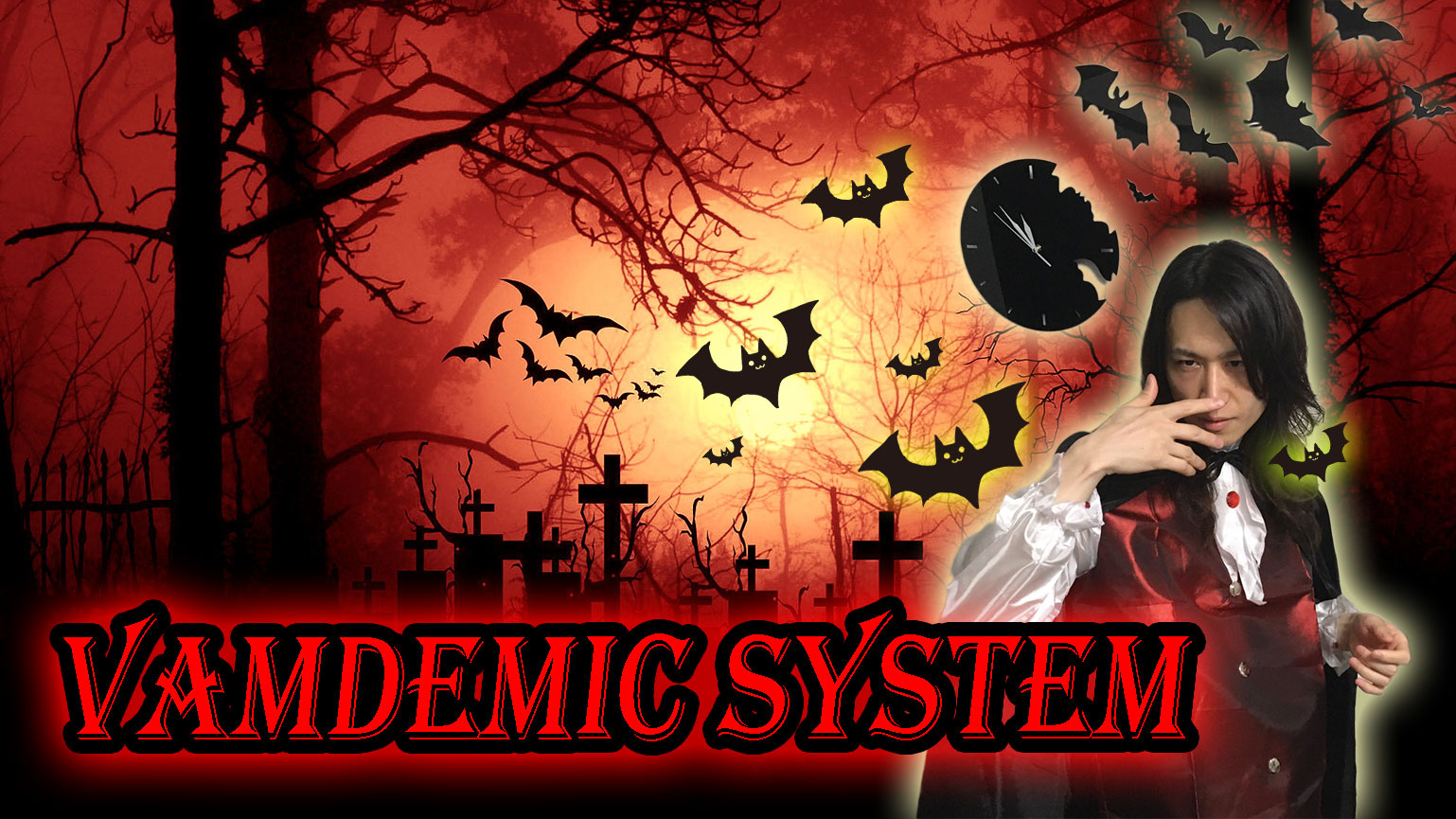
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 |
version: "3" services: postgres: image: postgres container_name: postgres ports: - 5432:5432 environment: - POSTGRES_USER=root - POSTGRES_PASSWORD=password tty: true restart: always user: root volumes: - ./init:/docker-entrypoint-initdb.d - /etc/localtime:/etc/localtime:ro pgweb: image: sosedoff/pgweb container_name: pgweb ports: - "8081:8081" environment: - DATABASE_URL=postgres://root:password@postgres:5432/testdb?sslmode=disable links: - postgres:postgres restart: always depends_on: - postgres |
1 |
docker-compose up -d |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
package main import ( "database/sql" "fmt" _ "github.com/lib/pq" ) const ( // Initialize connection constants. HOST = "127.0.0.1" DATABASE = "testdb" USER = "root" PASSWORD = "password" ) func checkError(err error) { if err != nil { panic(err) } } func main() { // Initialize connection string. var connectionString string = fmt.Sprintf("host=%s user=%s password=%s dbname=%s sslmode=disable", HOST, USER, PASSWORD, DATABASE) // Initialize connection object. db, err := sql.Open("postgres", connectionString) checkError(err) err = db.Ping() checkError(err) fmt.Println("Successfully created connection to database") // Drop previous table of same name if one exists. _, err = db.Exec("DROP TABLE IF EXISTS inventory;") checkError(err) fmt.Println("Finished dropping table (if existed)") // Create table. _, err = db.Exec("CREATE TABLE inventory (id serial PRIMARY KEY, name VARCHAR(50), quantity INTEGER);") checkError(err) fmt.Println("Finished creating table") // Insert some data into table. sql_statement := "INSERT INTO inventory (name, quantity) VALUES ($1, $2);" _, err = db.Exec(sql_statement, "banana", 150) checkError(err) _, err = db.Exec(sql_statement, "orange", 154) checkError(err) _, err = db.Exec(sql_statement, "apple", 100) checkError(err) fmt.Println("Inserted 3 rows of data") } |
1 |
go run main.go |
1 |
psql -h localhost -U root -d postgres -p 5432 |
1 |
\l |
1 |
\c testdb |
1 |
select current_database(); |
1 2 3 4 5 6 |
testdb=# select * from inventory; id | name | quantity ----+--------+---------- 1 | banana | 150 2 | orange | 154 3 | apple | 100 |
https://qiita.com/hiro9/items/e6e41ec822a7077c3568
https://docs.microsoft.com/ja-jp/azure/postgresql/connect-go