【Go】go-json-restモジュールを使って簡単にAPIサーバを作る
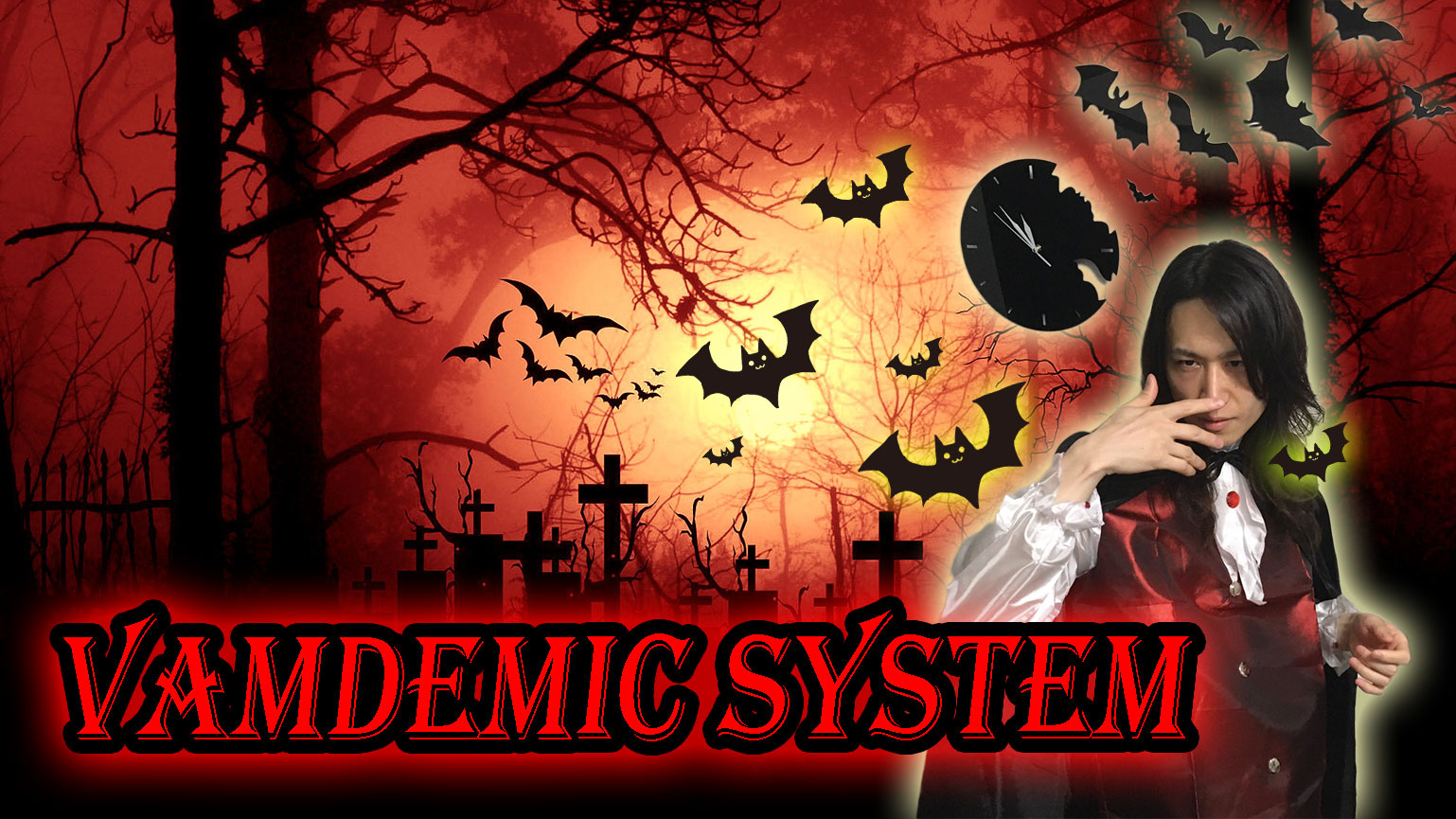
https://github.com/ant0ine/go-json-rest
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
package main import ( "github.com/ant0ine/go-json-rest/rest" "log" "net/http" ) func main() { api := rest.NewApi() api.Use(rest.DefaultDevStack...) api.SetApp(rest.AppSimple(func(w rest.ResponseWriter, r *rest.Request) { w.WriteJson(map[string]string{"Body": "Hello World!"}) })) log.Fatal(http.ListenAndServe(":8080", api.MakeHandler())) } |
1 2 3 4 5 6 7 8 9 10 |
yuta:~ $ curl -i http://127.0.0.1:8080/ HTTP/1.1 200 OK Content-Type: application/json; charset=utf-8 X-Powered-By: go-json-rest Date: Sun, 01 Nov 2020 00:54:41 GMT Content-Length: 28 { "Body": "Hello World!" }yuta:~ $ |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 |
package main import ( "github.com/ant0ine/go-json-rest/rest" "log" "net/http" "sync" ) func main() { api := rest.NewApi() api.Use(rest.DefaultDevStack...) router, err := rest.MakeRouter( rest.Get("/countries", GetAllCountries), rest.Post("/countries", PostCountry), rest.Get("/countries/:code", GetCountry), rest.Delete("/countries/:code", DeleteCountry), ) if err != nil { log.Fatal(err) } api.SetApp(router) log.Fatal(http.ListenAndServe(":8080", api.MakeHandler())) } type Country struct { Code string Name string } var store = map[string]*Country{} var lock = sync.RWMutex{} func GetCountry(w rest.ResponseWriter, r *rest.Request) { code := r.PathParam("code") lock.RLock() var country *Country if store[code] != nil { country = &Country{} *country = *store[code] } lock.RUnlock() if country == nil { rest.NotFound(w, r) return } w.WriteJson(country) } func GetAllCountries(w rest.ResponseWriter, r *rest.Request) { lock.RLock() countries := make([]Country, len(store)) i := 0 for _, country := range store { countries[i] = *country i++ } lock.RUnlock() w.WriteJson(&countries) } func PostCountry(w rest.ResponseWriter, r *rest.Request) { country := Country{} err := r.DecodeJsonPayload(&country) if err != nil { rest.Error(w, err.Error(), http.StatusInternalServerError) return } if country.Code == "" { rest.Error(w, "country code required", 400) return } if country.Name == "" { rest.Error(w, "country name required", 400) return } lock.Lock() store[country.Code] = &country lock.Unlock() w.WriteJson(&country) } func DeleteCountry(w rest.ResponseWriter, r *rest.Request) { code := r.PathParam("code") lock.Lock() delete(store, code) lock.Unlock() w.WriteHeader(http.StatusOK) } |
1 2 3 4 5 6 7 8 9 10 11 12 |
yuta:~ $ curl -i -H 'Content-Type: application/json' \ > -d '{"Code":"FR","Name":"France"}' http://127.0.0.1:8080/countries HTTP/1.1 200 OK Content-Type: application/json; charset=utf-8 X-Powered-By: go-json-rest Date: Sun, 01 Nov 2020 00:59:38 GMT Content-Length: 38 { "Code": "FR", "Name": "France" }yuta:~ $ |
1 2 3 4 5 6 7 8 9 10 11 |
yuta:~ $ curl -i http://127.0.0.1:8080/countries/FR HTTP/1.1 200 OK Content-Type: application/json; charset=utf-8 X-Powered-By: go-json-rest Date: Sun, 01 Nov 2020 01:01:02 GMT Content-Length: 38 { "Code": "FR", "Name": "France" }yuta:~ $ |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
yuta:~ $ curl -i -X DELETE http://127.0.0.1:8080/countries/FR HTTP/1.1 200 OK Content-Type: application/json; charset=utf-8 X-Powered-By: go-json-rest Date: Sun, 01 Nov 2020 01:01:35 GMT Content-Length: 0 yuta:~ $ curl -i http://127.0.0.1:8080/countries/FR HTTP/1.1 404 Not Found Content-Type: application/json; charset=utf-8 X-Powered-By: go-json-rest Date: Sun, 01 Nov 2020 01:01:41 GMT Content-Length: 35 { "Error": "Resource not found" }yuta:~ $ yuta:~ $ ^C |